Differentiating between a styled-component and a React component
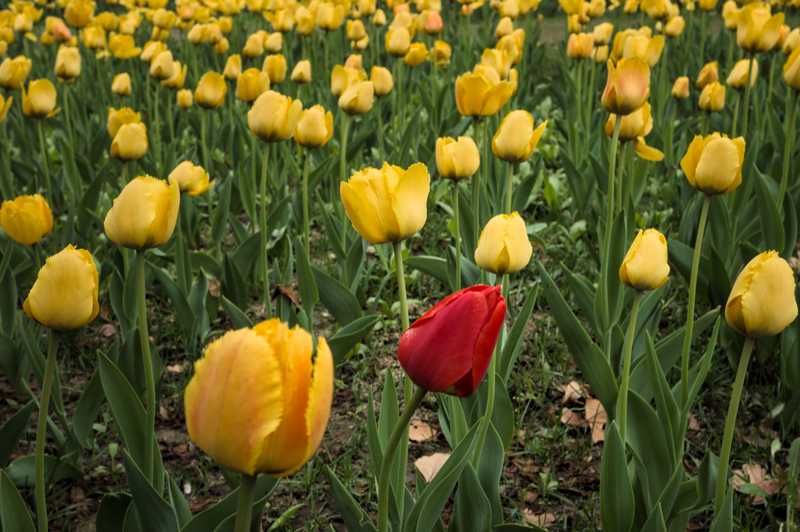
It can be irritating not knowing at first glance, if your component is a dumb style component or a React component. The only way to see it is to track down its origins. Some don’t mind but others do.
When we write vanilla CSS or something like CSS modules, we use JSX which looks like raw html tags and to style it we use the property className. This clearly helps us to see the difference between a dumb component and a React component:
<div className="App-header">
<Title>{title}</Title>
</div>
Here it’s clear that <Title>
is a React component, otherwise it would be:
<p className="title">{title}</p>
Here’s an example of where we don’t see the difference:
const ListItem = ({ title }) => (
<Container>
<Title>{title}</Title>
...
</Container>
)
###…Is <Title>
a styled-component?
const Title = styled.p`
color: white;
`
###…or is it a React component?
import React from "react"
const Title = ({ children }) => {
return <p>{children}</p>
}
export default Title
###we don’t know. ###I’ll talk about 2 ways to improve this
1. If you separate styles and logic
If you’ve chosen to keep all your styles in another file (like I talked about in Point #1 - article 2 of this series), you can do this little trick.
You create some styled-components in your style.js file.
export const Paragraph = styled.button`
color: red;
font-size: 14px;
`
export const Button = styled.button`
color: black;
font-size: 14px;
`
Then you could import all those styled components into your React component as such:
import * as Styled from "./styled.js"
Then in your React component you could name your button
<Styled.Paragraph> my paragraph </Styled.Paragraph>
<Styled.Button> my button </Styled.Button>
This is a pretty elegant way of prefixing your dumb styled components.
2. Add prefixes, Styled-, S- or $-
Here we’re using some kind of Hungarian notation. If you’ve heard about it before, chances are that you’ve only heard negative things about it and that you shouldn’t use it. Yet can be useful when used correctly.
Styled -
When you define the styled component, you can add the prefix “Styled-” like <StyledButton/>
, <StyledList />
etc. Like here. In this Github example the prefixing is however not being used for the same purposes. Here the prefixing only serves the purpose of preventing naming collision, so that the styled components won’t bear same name as the React components - which is also a pretty great side effect of what we’re doing here.
Another way to avoid name collision with the React component itself is to name the outermost element <Container>
which is not very descriptive. But if you prefix your styled-components, there’s no need to do that.
$ -
“Styled-” could become rather dull prefix to type out every time you need a styled-component, as it’s pretty long. Instead of that, you could use the dollar sign as a prefix
const $Button = styled.button``
and you could do the same for the props the SC can take, so
<$Button $color="green">My button</$button>
S -
If you’re using Typescript and you use prefixes you could use S- for styled components, I- for interfaces, etc.
So now with some simple prefixing we can make our styled-components more legible which will save us some time guessing which components are pure React components and which are not!
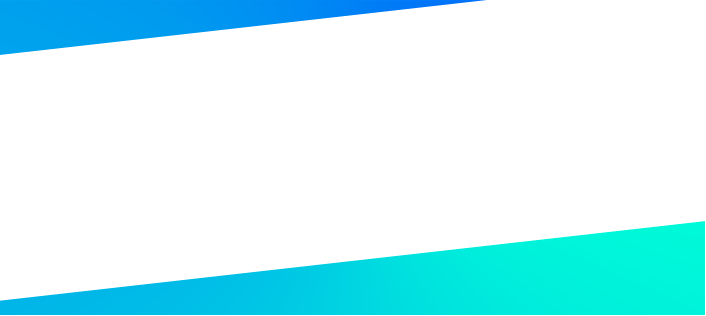